Basic Concept
Spring Setup
Spring Boot
Component Scanning in Spring with Example
In Spring, component scanning is a process that allows the Spring container to automatically discover and register beans within a specified package or set of packages. This is especially useful when you have a large application with many beans, as it eliminates the need to manually configure each one in an XML file or Java configuration class.
Spring Component
In Spring, a component is a general term for a managed object, which can be a service, repository, controller, or any other class you want to be managed by the Spring container. Components are typically annotated with @Component or one of its specialized annotations (@Service, @Repository, @Controller) depending on their role in the application.
Example of a Component
@Component
public class MyService {
// ...
}
Component Scanning
To enable component scanning, you need to specify a base package or packages from which the Spring container will start searching for components. This is usually done in the Spring configuration file or a Java configuration class.
Top premium Spring Boot interview questions in 2024 asked regularly (Free)
XML Configuration
In an XML-based configuration, you use the <context:component-scan> element to enable component scanning:
<context:component-scan base-package="com.example" />
This tells Spring to scan the package com.example and its subpackages for components.
Java Annotation Based Configuration
In a Java-based configuration, you use the @ComponentScan annotation on a configuration class:
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// ...
}
This annotation replaces the need for XML-based component scanning.
Combining with Stereotype Annotations
When component scanning is enabled, you can use stereotype annotations (@Component, @Service, @Repository, @Controller)to indicate the role of a class. Spring will automatically detect and register these annotated classes as beans.
Service annotaion example
@Service
public class MyService {
// ...
}
Repository annotaion example
@Repository
public class MyRepository {
// ...
}
Controller annotaion example
@Controller
public class MyController {
// ...
}
Benefits of Component Scanning
- Reduced Configuration Component scanning reduces the need for manual bean registration, making your configuration files cleaner and more concise.
- Improved Maintainability When adding or removing components, you don't have to update the configuration file, reducing the risk of configuration errors.
- Automatic Dependency Injection Annotated components become candidates for dependency injection, simplifying the wiring process.
- Flexibility Component scanning allows for easier reorganization of your codebase, as long as you maintain proper package structure and annotations.
Component scanning is a powerful feature in Spring that simplifies the process of managing beans and encourages the use of annotations for configuration. It is widely used in modern Spring applications to achieve cleaner and more maintainable code.
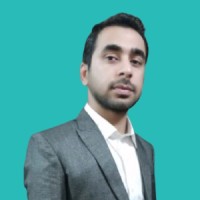
Aamir Shayan
Software Engineer