Hibernate Example Using XML in Intellij Idea and Eclipse
We already saw and created application using hibernate annotations in Eclipse now we are creating application using xml configuration in Intellij Idea. We will launch Intellij IDE and create new project.
Eclipse Setup and Installation
Follow the steps in this link to create new project and install Eclipse IDE
Before to proceed you have to make sure that you have setup hibernate in Eclipse using above link, then create BookEntity class in src/main/javadirectory.
BookEntity.java
public class BookEntity { private Integer id; private String bookName; private Integer authorId; public BookEntity() {} // Getters and Setters }
The no-argument constructor is required by all persistence classes because hibernate creates the object instances per reflection. In this case private constructor prevents creation of book without information.
Create mapping file for persistent class
A mapping file is required by hibernate for the entities to map the right field to the right column in the database. The mapping information is always stored in .hmb.xml file. In this Example we will create Book.hmb.xml.
Book.hmb.xml
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="entity"> <class name="BookEntity" table="book"> <id name="id" type="java.lang.Integer"/> <property name="bookName" column="book_name"/> <property name="authorId" column="author_id"/> </class> </hibernate-mapping>
Create hibernate configuration file
The configuration file has all the information for the database such as connection_url, driver_class, username, password and more. The hbm2ddl.auto property is used foe creating the table in the database automatically. We will dive deep learning about Dialect class in upcoming tutorials.
hibernate.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate_db</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password">root</property> <property name="hibernate.connection.pool_size">1</property> <property name="hibernate.current_session_context_class">thread</property> <property name="hibernate.show_sql">true</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <mapping resource="Book.hbm.xml"/> </session-factory> </hibernate-configuration>
Paste the following code in main method to execute the code
public static void main(String[] arg) { try { StandardServiceRegistry ssr = new StandardServiceRegistryBuilder().configure("hibernate.cfg.xml").build(); Metadata meta = new MetadataSources(ssr).getMetadataBuilder().build(); SessionFactory factory = meta.getSessionFactoryBuilder().build(); Session session = factory.openSession(); session.beginTransaction(); BookEntity book = new BookEntity(); book.setBookName("C++ Book"); book.setAuthorId(1); book.setId(2); session.save(book); session.getTransaction().commit(); System.out.println("Data Saved"); } catch (Exception e) { e.printStackTrace(); } }
Here is file structure in Intellij Idea
After executting application you will see logs
Data Store in Book Table in database
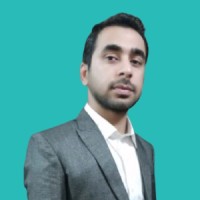
Aamir Shayan
Software Engineer