Basic Concept
Spring Setup
Spring Boot
Mastering REST APIs in Spring Boot: A Comprehensive Guide for Quick and Efficient Development - Part 2
Welcome to the Spring Boot REST API development series. This series consists of several parts. In part 1 we created a static API, In this part we will add business layer to it.
In a Spring Boot application, the business layer is where the core logic of your application resides. It's responsible for implementing the business rules, processing data, and interacting with the data access layer. The business layer typically includes services that handle the application's business logic and orchestrate interactions between different components.
Let's extend the existing example by adding a business layer for managing students. We'll create a StudentService Interface and a StudentServiceImpl class that will handle the business logic related to students. We will discuss them in details separately.
Mastering Spring Boot: Building a Static REST API - Part 1
Mastering Spring Boot: Adding Data Access Layer - Part 3
Mastering Spring Boot: Building CRUD REST API - Part 4
Mastering Spring Boot: Exception Handling - Part 5
Following snippet is showing the project structure, file and folder tree
Project Structure
Create StudentService.java interface
import java.util.List;
public interface StudentService {
List<Student> getAllStudents();
}
Create StudentServiceImpl.java class
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class StudentServiceImpl implements StudentService{
private final StudentRepository studentRepository;
StudentServiceImpl(StudentRepository studentRepository){
this.studentRepository = studentRepository;
}
@Override
public List<Student> getAllStudents() {
return studentRepository.findAll();
}
}
@Service annotation marks this class as a Spring service, allowing it to be automatically discovered and injected into other components.
The methods in this class represent common operations related to students, such as retrieving or getting all students. In a real-world scenario, these such method would interact with a database or another data source.
Modify existing StudentController.java class
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@RequestMapping("/api")
public class StudentController {
private final StudentService studentService;
StudentController(StudentService studentService){
this.studentService = studentService;
}
@GetMapping("/students")
public List<Student> getAllStudents(){
return studentService.getAllStudents();
}
}
The StudentController now uses an instance of StudentService to handle the business logic related to students.
Constructor-injection is used to inject the StudentService into the controller.
Student.java
public class Student {
private Integer id;
private String name;
private String email;
public Student(Integer id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
public Integer getId() {
return id;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
By introducing a business layer, you separate concerns in your application. The controller is responsible for handling HTTP requests and responses, while the service deals with the business logic. This separation enhances maintainability, testability, and overall code organization. The service layer can also be easily replaced or extended without affecting the controller or other components.
Check API response
Once your application starts successfully then hit this URL to get the response
http://localhost:8080/api/students
API response
[
{
"id": 1,
"name": "Aamir",
"email": "aamir@aamir.com"
},
{
"id": 2,
"name": "Shayan",
"email": "shayan@shayan.com"
},
{
"id": 3,
"name": "Kashif",
"email": "kashif@kashif.com"
}
]
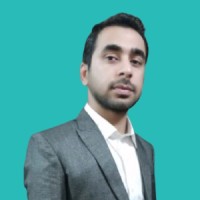
Aamir Shayan
Software Engineer