Java Core Interview Questions
SQL Basic Interview Questions
Spring Must Know Interview Questions
Spring Boot Must Know Interview Questions
Unveiling the Spring MVC Interview: Your Ultimate Guide to Top Questions and Expert Answers - Chapter 4
Prepare for your Java developer interview with confidence! Explore the essential Spring MVC interview questions in our comprehensive guide. Learn how to tackle common challenges, demonstrate your expertise, and secure your dream job in Java development.
What is DispatcherServlet?
DispatcherServlet acts as the central "front controller" for Spring MVC applications. It is a Servlet that receives all incoming HTTP requests and delegates them to appropriate controller classes. The DispatcherServlet is responsible for identifying the appropriate handler method for each request and invoking it, ensuring that the request is processed correctly.
The following example of the Java configuration which is responsible for registering and initializing the DispatcherServlet, and it is auto-detected by the Servlet container.
public class MyWebApplicationInitializer implements
WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) {
// Load Spring web application configuration
AnnotationConfigWebApplicationContext context = new
AnnotationConfigWebApplicationContext();
context.register(AppConfig.class);
// Create and register the DispatcherServlet
DispatcherServlet servlet = new DispatcherServlet(context);
ServletRegistration.Dynamic registration =
servletContext.addServlet("app", servlet);
registration.setLoadOnStartup(1);
registration.addMapping("/app/*");
}
}
What is @Controller annotation in spring?
The @Controller annotation is a Spring stereotype annotation that indicates that a class serves as a web controller. It is primarily used in Spring MVC applications to mark classes as handlers for HTTP requests. When a class is annotated with @Controller, it can be scanned by the Spring container to identify its methods as potential handlers for specific HTTP requests.
Spring MVC provides an annotation-based programming model where @Controller and @RestController components use annotations to express request mappings, request input, exception handling, and more. Annotated controllers have flexible method signatures and do not have to extend base classes nor implement specific interfaces. The following example shows a controller defined by annotations:
@Controller
public class HelloController {
@GetMapping("/hello")
public String handle(Model model) {
model.addAttribute("message", "Hello World!");
return "index";
}
}
How Controller maps appropriate methods to incoming request?
You can use the @RequestMapping annotation to map requests to controller methods. It has various attributes to match by URL, HTTP method, request parameters, headers, and media types. You can use it at the class level to express shared mappings or at the method level to narrow down to a specific endpoint mapping.Request Mapping Process:
There are also HTTP method specific shortcut variants of @RequestMapping:
@GetMapping
@PostMapping
@PutMapping
@DeleteMapping
@PatchMapping
Request Reception:
An incoming HTTP request received by DispatcherServlet, containing the request URI, HTTP method (GET, POST, PUT, DELETE, etc.) and request parameters.
Mapping Lookup:
The DispatcherServlet utilizes a HandlerMapping component to lookup the appropriate handler method for the received request. The HandlerMapping maintains a registry of mappings between request patterns and handler methods.
Pattern Matching:
The HandlerMapping compares the request URI and HTTP method against the registered request patterns. It uses pattern matching rules to identify the most specific matching pattern.
Handler Method Identification:
Once the matching pattern is identified, the HandlerMapping retrieves the corresponding handler method from its registry. This handler method is the one responsible for handling the incoming request.
Method Invocation:
The DispatcherServlet invokes the identified handler method, passing the request object as an argument. The handler method processes the request's logic and generates an appropriate response.
Response Handling:
After the handler method completes its execution, the DispatcherServlet receives the generated response object. It prepares the response by setting appropriate headers and content, and sends the response back to the client.
This is the sample program,
@RestController
@RequestMapping("/persons")
class PersonController {
@GetMapping("/{id}")
public Person getPerson(@PathVariable Long id) {
// ...
}
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
public void add(@RequestBody Person person) {
// ...
}
}
What is the difference between @Controller and @RestController in Spring MVC?
@Controller: Designates a class as a controller for traditional MVC applications.
Methods typically return a view name (String), which is resolved by a view resolver to render a view (e.g., an HTML page).
Can also return void, indicating that the view name is the same as the request path.
Can use @ResponseBody on individual methods to return data directly (e.g., JSON), but this isn't the
default behavior.
@RestController: Specialized controller for building RESTful web services.
Implicitly applies @ResponseBody to all handler methods, so they return data directly in the
response body, typically in JSON or XML format.
No need for view resolution or manual settings for returning data.
Simplifies the development of REST APIs.
When to Use Each: Use @Controller for traditional web applications that focus on rendering views and returning HTML content.
Use @RestController for building RESTful APIs that primarily return data in formats like JSON or XML.
Example:
@Controller
public class MyController {
@GetMapping("/hello")
public String hello() {
return "hello"; // Returns the view name "hello"
}
}
@RestController
public class MyRestController {
@GetMapping("/greeting")
public String greeting() {
return "Hello, World!"; // Returns "Hello, World!" as JSON or XML
}
}
Difference between @Requestparam and @Pathparam annotation?
@Requestparam: You can use the @RequestParam annotation to bind Servlet request parameters (that is, query parameters or form data) to a method argument in a controller. Here code example,
@Controller
@RequestMapping("/pets")
public class EditPetForm {
@GetMapping
public String setupForm(@RequestParam("petId") int petId, Model
model) {
Pet pet = this.clinic.loadPet(petId);
model.addAttribute("pet", pet);
return "petForm";
}
}
Using @RequestParam we are binding petId. By default, method parameters that use this annotation are required, but you can specify that a method parameter is optional by setting the @RequestParam annotation’s required flag to false or by declaring the argument with an java.util.Optional wrapper.
@Pathparam
Function: It allows you to map variables from the request URI path to method parameters in your Spring controller. This gives you a cleaner and more flexible way to handle dynamic data in your
API.
Usage: You place the @Pathparam annotation on a method parameter. Inside the annotation, you specify the name of the variable in the URI path that should be bound to the parameter.
What is session scope used for?
session scope is a way of managing the lifecycle of objects that are bound to a specific HTTP session. When an object is created in session scope, it is stored in the session and is accessible to all requests that belong to the same session. This can be useful for storing user-specific information or maintaining state across multiple requests.
What is the most common Spring MVC annotations?
Controller Annotations:
@Controller: Designates a class as a controller, responsible for handling HTTP requests and rendering responses.
@RestController: A specialized version of @Controller that implicitly adds
@ResponseBody to all handler methods, indicating that they should directly write data to the response body, often in JSON or XML format.
Request Mapping Annotations:
@RequestMapping: Maps web requests to specific controller methods based on URL patterns, HTTP methods (GET, POST, PUT, DELETE, etc.), request parameters, and headers. @GetMapping, @PostMapping, @PutMapping, @DeleteMapping, @PatchMapping: Convenient shortcuts for mapping specific HTTP methods.
@PathVariable: Binds a method parameter to a path segment variable in the URL. @RequestParam: Binds a method parameter to a query parameter in the request URL.
Data Binding Annotations:
@ModelAttribute: Populates a model attribute with an object, making it available to the view.
@RequestParam: Binds a request parameter to a method argument.
@RequestHeader: Binds a request header to a method argument.
@RequestBody: Maps the request body to a method argument, often used for JSON or XML data.
Response Handling Annotations:
@ResponseBody: Indicates that the method's return value should be written directly to the response body, bypassing view resolution.
@ResponseStatus: Sets the HTTP status code of the response.
Exception Handling Annotations:
@ExceptionHandler: Defines a method to handle exceptions of a specific type, providing a centralized way to manage errors.
Other Useful Annotations:
@SessionAttribute: Accesses a session attribute.
@ModelAttribute: Adds an attribute to the model for all handler methods in a controller.
@InitBinder: Customizes data binding and validation for a controller.
@CrossOrigin: Enables cross-origin requests for a controller or specific handler methods.
Can singleton bean scope handle multiple parallel requests?
A singleton bean in Spring has a single instance that is shared across all requests, regardless of the number of parallel requests. This means that if two requests are processed simultaneously, they will share the same bean instance and access to the bean's state will be shared among the requests. However, it's important to note that if the singleton bean is stateful, and the state is shared among requests, this could lead to race conditions and other concurrency issues. For example, if two requests are trying to modify the same
piece of data at the same time, it could lead to data inconsistencies.
To avoid these issues, it's important to make sure that any stateful singleton beans are designed to be thread-safe. One way to do this is to use synchronization or other concurrency control mechanisms such as the synchronized keyword, Lock or ReentrantLock classes, or the @Transactional annotation if the bean is performing database operations.
On the other hand, if the singleton bean is stateless, it can handle multiple parallel requests without any issues. It can be used to provide shared functionality that doesn't depend on the state of the bean. In conclusion, a singleton bean can handle multiple parallel requests, but it's important to be aware of the state of the bean and to ensure that it's designed to be thread-safe if it has shared state.
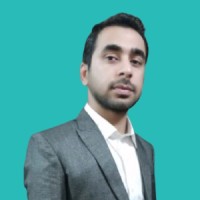
Aamir Shayan
Software Engineer