Collection Set Mapping in Hibernate using XML
When there is collection type Set<> in Persistent class we can map it using set tag in mapping file. The set tag does not require index tag. The main difference between list and set is that set allows to store unique values only.
Set mapping using set tag
The code bellow is used for mapping set in mapping file.
<set name="orders" cascade="all"> <!--Collection tag--> <key column="customer_frk_id" not-null="true" on-delete="cascade" update="true" unique="false" /> <one-to-many class="Orders"/> <!--relation--> </set>
Collection Example of mapping set in hibernate
In this example we are will see complete working example of collection mapping by set.
Create persistent class
Persistent class will contain properties of the entity and Set
Customer.java
public class Customer { private Integer customerId; private String customerName; private Set<Orders> orders; // Collection type // Getters and Setters }
Orders.java
public class Orders { private Integer orderId; private String orderDetail; // Getters and Setters }
Create mapping files for persistent classes
Following are the mapping files for persistent classes.
customer.hbm.xml
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 5.3//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="entityonetomany"> <class name="Customer"> <!--Class tag--> <id name="customerId"> <generator class="increment"></generator> </id> <property name="customerName" ></property> <set name="orders" cascade="all"> <!--Collection tag--> <key column="customer_frk_id" not-null="true" on-delete="cascade" update="true" unique="false" /> <one-to-many class="Orders"/> <!--relation--> </set> </class> </hibernate-mapping>
order.hbm.xml
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 5.3//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="entityonetomany"> <class name="Orders"> <id name="orderId"> <generator class="increment"></generator> </id> <property name="orderDetail"></property> </class> </hibernate-mapping>
Create hibernate configuration file
The configuration file contains the information about the mapping files and database
hibernate.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate_db</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password">root</property> <property name="hibernate.connection.pool_size">1</property> <property name="hibernate.current_session_context_class">thread</property> <property name="hibernate.show_sql">true</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</property> <property name="hibernate.hbm2ddl.auto">create</property> <mapping resource="customer.hbm.xml"/> <mapping resource="order.hbm.xml"/> </session-factory> </hibernate-configuration>
Create Hibernate Util Class
Create hibernateutil class for providing single session factory object for creating session in main class.
HibernateUtil.java
import org.hibernate.SessionFactory; import org.hibernate.boot.Metadata; import org.hibernate.boot.MetadataSources; import org.hibernate.boot.registry.StandardServiceRegistry; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; public class HibernateUtil { private HibernateUtil() { } private static SessionFactory sessionFactory; public static synchronized SessionFactory getInstnce() { if (sessionFactory == null) { StandardServiceRegistry ssr=new StandardServiceRegistryBuilder().configure("hibernate.cfg.xml").build(); Metadata meta=new MetadataSources(ssr).getMetadataBuilder().build(); sessionFactory = meta.getSessionFactoryBuilder().build(); } return sessionFactory; } }
Create class for main method
Create a class and main method that will store data into database and retreive it.
MyMain.java
public class MyClass { public static void main(String[] arg) { try { SessionFactory sessionFactory = HibernateUtil.getInstnce(); Session session = sessionFactory.openSession(); Customer customer = new Customer(); customer.setCustomerName("Aamir"); Set<Orders> orders = new HashSet<Orders>(); Orders order1 = new Orders(); order1.setOrderDetail("One Pasta"); orders.add(order1); Orders order2 = new Orders(); order2.setOrderDetail("One Rice"); orders.add(order2); customer.setOrders(orders); Transaction transaction = session.beginTransaction(); session.save(customer); // Saving customer and orders /*##################### Retrieving Data ################################*/ TypedQuery query = session.createQuery("from Customer c"); // Retrieving customer and orders from database List<Customer> list = query.getResultList(); Iterator<Customer> itr = list.iterator(); while (itr.hasNext()) { customer = itr.next(); System.out.println(customer.getCustomerId() + " " + customer.getCustomerName() ); for (Orders ord: customer.getOrders()) { System.out.println(ord.getOrderId() + " " + ord.getOrderDetail()); } } transaction.commit(); session.close(); sessionFactory.close(); } catch (Exception e) { e.printStackTrace(); } } }
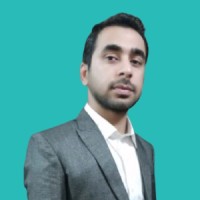
Aamir Shayan
Software Engineer